Effective error handling ensures your site delivers a stable and reliable browsing experience.
No website is perfect. Occasional glitches and delays are inevitable. But the true measure of a website’s quality is how it manages errors and exceptions to prevent them from ruining a website visitor’s experience.
Strategic error handling is a safeguard in these situations, enabling swift intervention to solve problems as they arise. This proactive approach lets you minimize the potential for disruption and strengthen user trust by demonstrating a commitment to a seamless and enjoyable browsing experience.
Understanding error handling
Every action a user takes on a website — like clicking links or submitting forms — triggers a background program. This program, or code, dictates how the site responds to user inputs, displays content, and manages navigation. But not every interaction goes as expected. Visitors might enter data the system doesn’t anticipate, or server issues could momentarily hinder site functionality, leading to potential errors and exceptions.
Effective error handling means identifying, analyzing, and fixing these issues to maintain smooth website operation. This includes managing exceptions to prevent them from halting programs and preventing your website from showing detailed error messages and sensitive information, minimizing cyberthreat risk. By concealing this information, error handling helps you maintain operational integrity and bolster your site’s security measures so you can provide a seamless and safe visitor experience.
What’s an error (and is it the same as an exception)?
An error is any unexpected situation during a program’s execution that might stem from logical mistakes or system failures. Imagine you create a website form to collect user feedback. The form includes a script that validates email addresses to ensure it follows a standard email format, such as user@website.com. But due to a logical error, the script incorrectly validates email addresses that lack an “@” symbol, allowing visitors to submit incomplete and invalid addresses.
Exceptions are a subset of errors that specifically interrupt the normal flow of program instructions, like when a program attempts to access a nonexistent file. If your website dynamically loads content from an external file or database when a user selects a specific option, an exception might occur if that file is missing or deleted. Since the expected resource is unavailable, the program encounters an exception, disrupting its intended operation.
3 common error types
Whether you’re customizing a checkout flow or setting up feedback forms, understanding error types is crucial to fixing them. Here are three common types.
1. Logic error
Logic errors stem from flaws in a program’s design or implementation that lead to unintended behavior without immediate system crashes. Consider a scenario in which the task is to calculate the average from a list of numbers. But due to a logic error, the code multiplies these numbers instead of summing them up. Because your code’s syntax is correct, logic errors won’t typically trigger flags during compilation or execution but will often manifest through abnormal program behaviors.
2. Compile-time errors
These errors arise when a program called a compiler translates human-readable code into a machine-executable format. This compilation process checks the code for syntax errors before producing an executable file.
Development languages like C, C++, Rust, and Java undergo a traditional compilation phase, while JavaScript, Python, and PHP are interpreted languages that don’t require compilation.
For compiled languages, compile-time errors occur during the compilation phase after you’ve written your code but before you execute the program. They usually consist of:
- Syntax issues where you get the “grammar” of your code wrong, such as typos or an unclosed parenthesis.
- Type mismatches where your code expects a number but you try to use a letter.
3. Runtime errors (exceptions)
Runtime errors, or exceptions, occur as the program runs and can lead to crashes if unhandled. Imagine an ecommerce site with a form for users to enter item quantities. If a user inputs a letter rather than a number and the code doesn’t safeguard against this input, attempting to calculate the total price will trigger a runtime error. This happens because the program expects a numerical value for multiplication but receives a letter instead.
Error handling configuration
Strategic error handling tailors its approach to the unique challenges of each error type.
Here are a few tips to resolve each error category effectively.
Handling logical errors
Debugging tools are indispensable for identifying logical programming errors. In JavaScript, for instance, leveraging the browser’s developer console and tools like Chrome DevTools and the Visual Studio Code debugger can be particularly helpful. These tools let you:
- Inspect variables. This feature lets you analyze variable values at different execution stages in real time, providing insights into your program’s state and helping you identify logical error sources.
- Set breakpoints. Breakpoints let you pause code execution at specified points to examine the program’s state and variables. This lets you isolate sections where issues might arise, making logical error detection faster.
- Step through code. Debugging tools enable line-by-line execution observation, showing you how the program changes variables and calls functions. By stepping through the code, you can witness the exact sequence of operations, track the values of variables, and identify discrepancies or unexpected behavior.
Handling compile-time errors
Interpreted or scripted languages, like JavaScript and Python, don’t go through traditional compilation phases or have explicit compile-time errors. For instance, JavaScript returns errors after catching them when your script loads or executes. In these languages, errors get identified and logged during the script’s load or execution. But compiled languages like C and Java halt the compilation process if the compiler detects errors and logs them for correction.
To manage compile-time errors, use an integrated development environment (IDE) or text editor with syntax checking and highlighting features. These resources, along with build tools and compilers, can pinpoint and help address compile-time errors by providing immediate feedback on potential issues. For instance, IDEs like Eclipse for Java or Visual Studio for C# offer comprehensive debugging tools that highlight syntax and logical errors in real time. They also suggest possible fixes, making maintaining clean, error-free custom code easier.
Handling runtime errors
When addressing runtime errors and exceptions, developers typically follow one of two approaches — error bubbling or immediate error handling.
Error bubbling lets an error rise, or “bubble,” from the initial occurrence point through nested functions until it reaches a higher level that lets the developer address it. This method depends on the hierarchical structure of the call stack to manage errors systematically.
But immediate error handling addresses errors at the point of occurrence and prevents them from affecting other application parts.
In both cases, you can use a try-catch block or error event listener to catch and process your error.
Try-catch blocks
A try-catch block is an error handler that exists in many programming languages. It’s a control flow statement that defines the sequence in which individual instructions or blocks of code are executed. These blocks encapsulate code that may trigger errors within a try segment, while the catch segment processes any errors that arise to prevent program crashes. This setup includes:
- Try block: Encases code that might trigger an error
- Catch block: Activates if an error occurs within the try block, with the error providing details about the issue
Here’s an example try-catch block syntax for JavaScript:
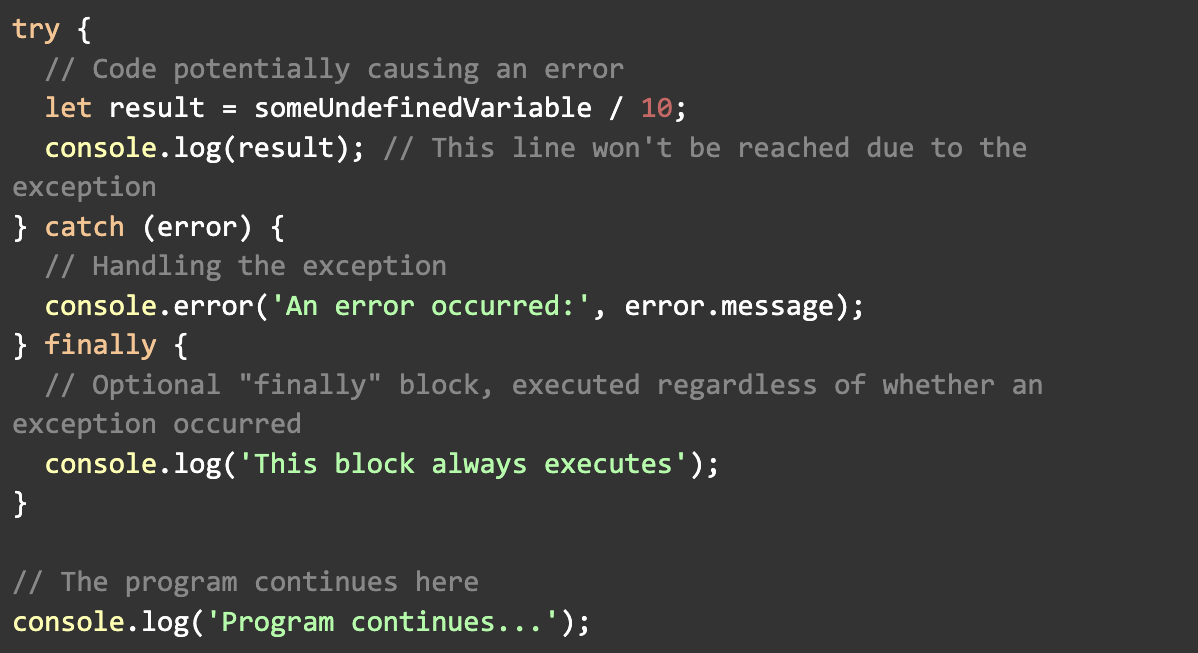
The try-catch block syntax varies for each language, so you’ll need to adapt the code to your needs.
Error event listener
Unlike the try-catch block, which deals with synchronous code execution, an error event listener allows developers to handle errors that occur asynchronously — outside the typical flow of synchronous code execution. These listeners are particularly effective in web development for handling errors from external resource loading and user interactions.
Employing error event listeners lets you intercept errors at a broader application level. This enables more effective error logging, debugging, and handling and ensures that asynchronous errors don’t interrupt the overall application flow.
Avoid errors with an automated testing suite
To complement the error-handling strategies discussed above, consider adopting automated testing suites.
These software tools automate code testing and reduce manual errors by simulating user interactions to detect issues early. By preventing errors from reaching production, automated suites streamline debugging and bolster application reliability, ultimately ensuring a seamless user experience. This approach embodies the DevOps shift left philosophy, which encourages early and frequent testing to identify errors before they escalate.
An effective testing suite includes the following key components.
Unit tests
Unit tests scrutinize individual code sections and functions to ensure each operates as expected. Because they look at each feature individually, these tests are great for identifying logic errors early in development.
As a best practice, you should write unit tests for each feature you create to allow for focused testing on component behavior without broader application contexts interfering.
Consider the earlier logic error example. After writing a feature, a developer would write a unit test that checks if the feature calculates the average from a list of numbers. If the feature incorrectly multiplies values instead, the unit test would highlight this discrepancy before the developer merges the code. This ensures errors are caught early.
Unit tests also excel at identifying runtime errors by testing various inputs, especially when dealing with unexpected data types. Consider a scenario where your number-averaging feature encounters nonnumeric data, like the letter “p,” as input. Should your form mistakenly accept this, a runtime error will occur. But by designing your feature only to accept specific data types, any inappropriate input triggers an error or warning message to the user. Unit testing ensures your feature robustly handles these edge cases to prevent runtime errors and maintain your application’s integrity.
Integration tests
Integration tests ensure seamless interaction between application components by simulating real-world scenarios where different parts work together. They identify interface issues and verify the correct data flow to prevent logic and runtime errors.
Consider a scenario where a runtime error occurs in the code responsible for database comment storage. An integration test could replicate submitting a comment through the web application, saving it to the database, and then displaying it on the website. This approach allows the test to identify any runtime errors early and prevent them from affecting the production environment. Integration tests can also help you troubleshoot code by highlighting error messages, unexpected outcomes, and any other signs that the process or program didn’t proceed as planned.
End-to-end tests
End-to-end (E2E) tests look at your entire application workflow, encompassing user interfaces, databases, and external integrations. They simulate user scenarios to uncover logic and runtime errors across the system.
While integration tests focus on interactions between different application components, E2E tests evaluate the entire application’s workflow from the user’s perspective. So while integration tests verify component compatibility, E2E tests validate the complete user experience.
For instance, in testing the graphical user interface (GUI) — the components users interact with — an E2E test might navigate through critical user flows, like logging in. It simulates clicks through the login sequence to ensure all forms work correctly, the systems accurately process and store user information, and the interface displays the proper information.
Enjoy error-free websites with Webflow
Understanding various error types and implementing strategies to manage or prevent them is essential. Webflow Enterprise offers a suite of analytics tools and integrations designed to simplify this process. These resources empower your team to identify, analyze, and address potential issues more efficiently, so you don’t have to worry about 502 bad gateway errors and security risks.
Explore Webflow Enterprise today and discover how our platform can revolutionize your online presence.
Webflow Enterprise gives your teams the power to build, ship, and manage sites collaboratively at scale.